GraphQL has its own language to write GraphQL Schemas: The GraphQL Schema Definition Language (SDL). SDL is simple and intuitive to use while being extremely powerful and expressive.
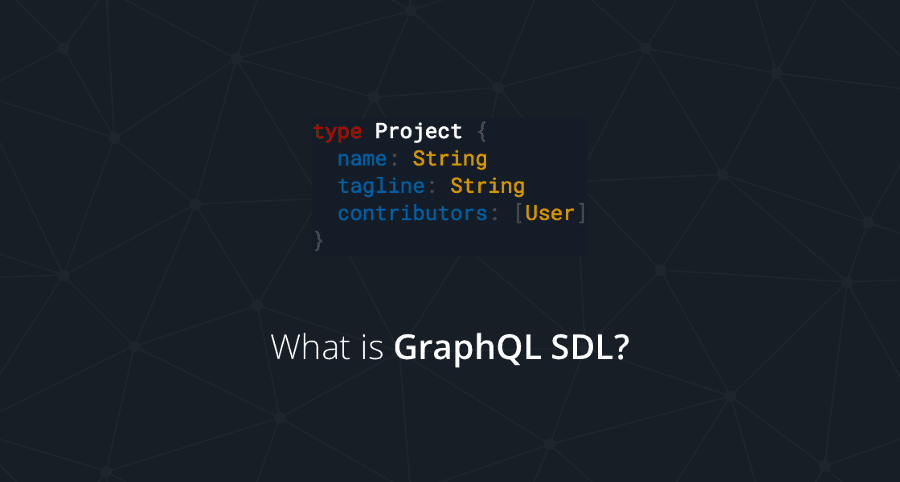
What is a GraphQL Schema Definition?
A GraphQL Schema Definition is the most concise way to specify a GraphQL schema. The syntax is well-defined and are part of the official GraphQL specification. Schema Definitions are sometimes referred to as IDL (Interface Definition Language) or SDL (Schema Definition Language).
The GraphQL schema for a blogging app could be specified like this:
The main components of a schema definition are the types and their fields. Additional information can be provided as custom directives like the @default
value specified for the likes
field.
Type
A type has a name and can implement one or more interfaces:
Field
A field has a name and a type:
The GraphQL spec defines some built-in scalar values but more can be defined by a concrete implementation. The built in scalar types are:
- Int
- Float
- String
- Boolean
- ID
In addition to scalar types, a field can use any other type defined in the schema definition.
Non-nullable fields are denoted by an exclamation mark:
Lists are denoted by square brackets:
Enum
An enum
is a scalar value that has a specified set of possible values:
Interface
In GraphQL an interface
is a list of fields. A GraphQL type must have the same fields as all the interfaces it implements and all interface fields must be of the same type.
Schema directive
A directive allows you to attach arbitrary information to any other schema definition element. Directives are always placed behind the element they describe:
Directives don’t have intrinsic meaning. Each GraphQL implementation can define their own custom directives that add new functionality.
GraphQL specifies built-in skip and include directives that can be used to include or exclude specific fields in queries, but these aren't used in the schema language.
Don’t miss the next post!
Sign up for the Prisma Newsletter